Adapter is a structural design pattern:
š¤š¤š¤š¤š¤
What is Adapter?
Adapter is a set of converters(USB-B to USB-C
, USB-A to USB-C
, HDMI to USB-C
).
Adapter makes it possible for the main device (Macbook
) to be connected to incompatible devices (<Printer>
, <Flash>
, <Monitor>
)
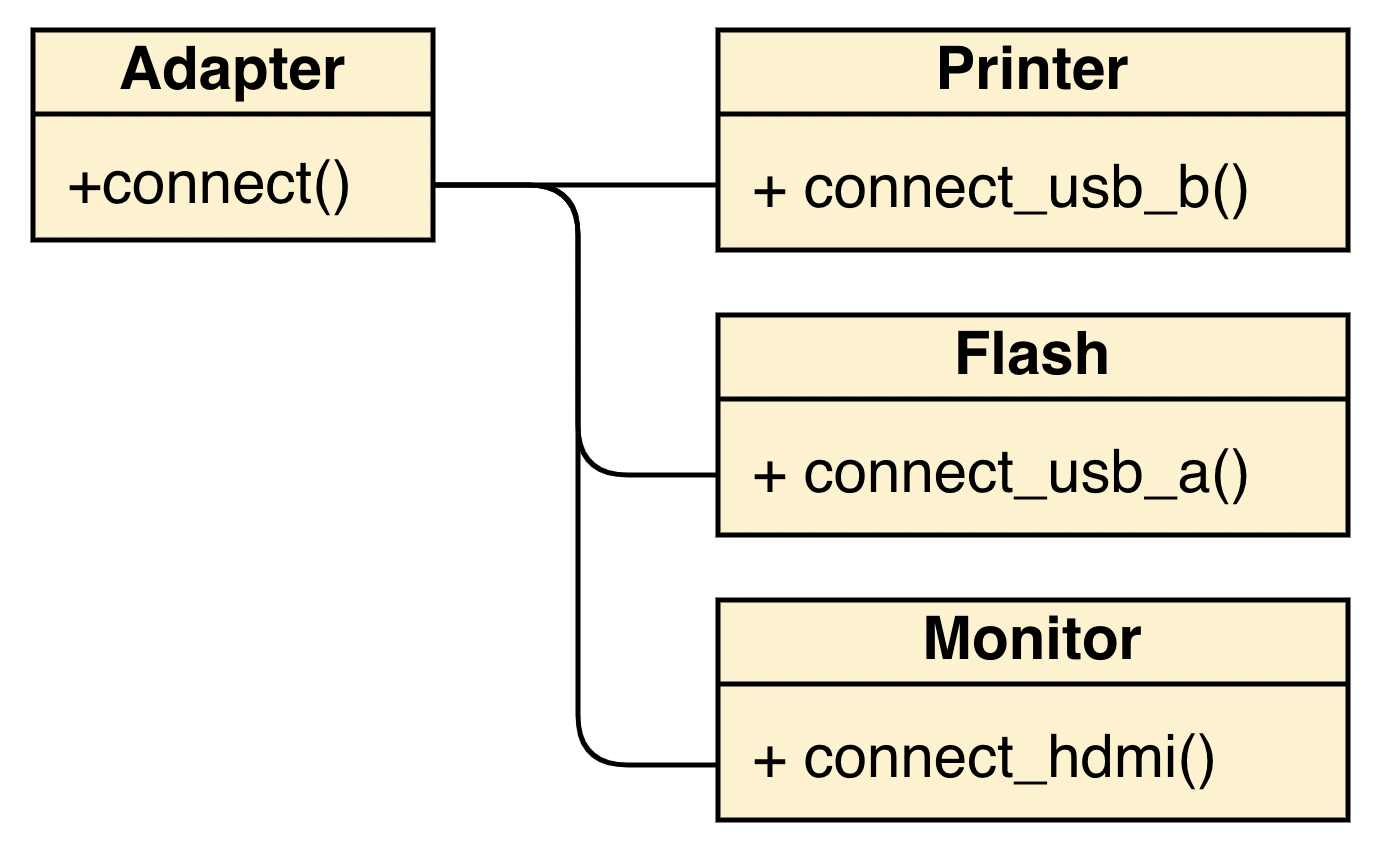
Why use Adapter?
It’s all in one converter, neat and clean
Use Adapter:

Question: I hate the Adapter, what are the alternatives?
Answer: You can buy separate converters(USB type B to type C
cable, USB type A to type C
cable, HDMI to type C
cable) for replacement!
Use Many Converters:
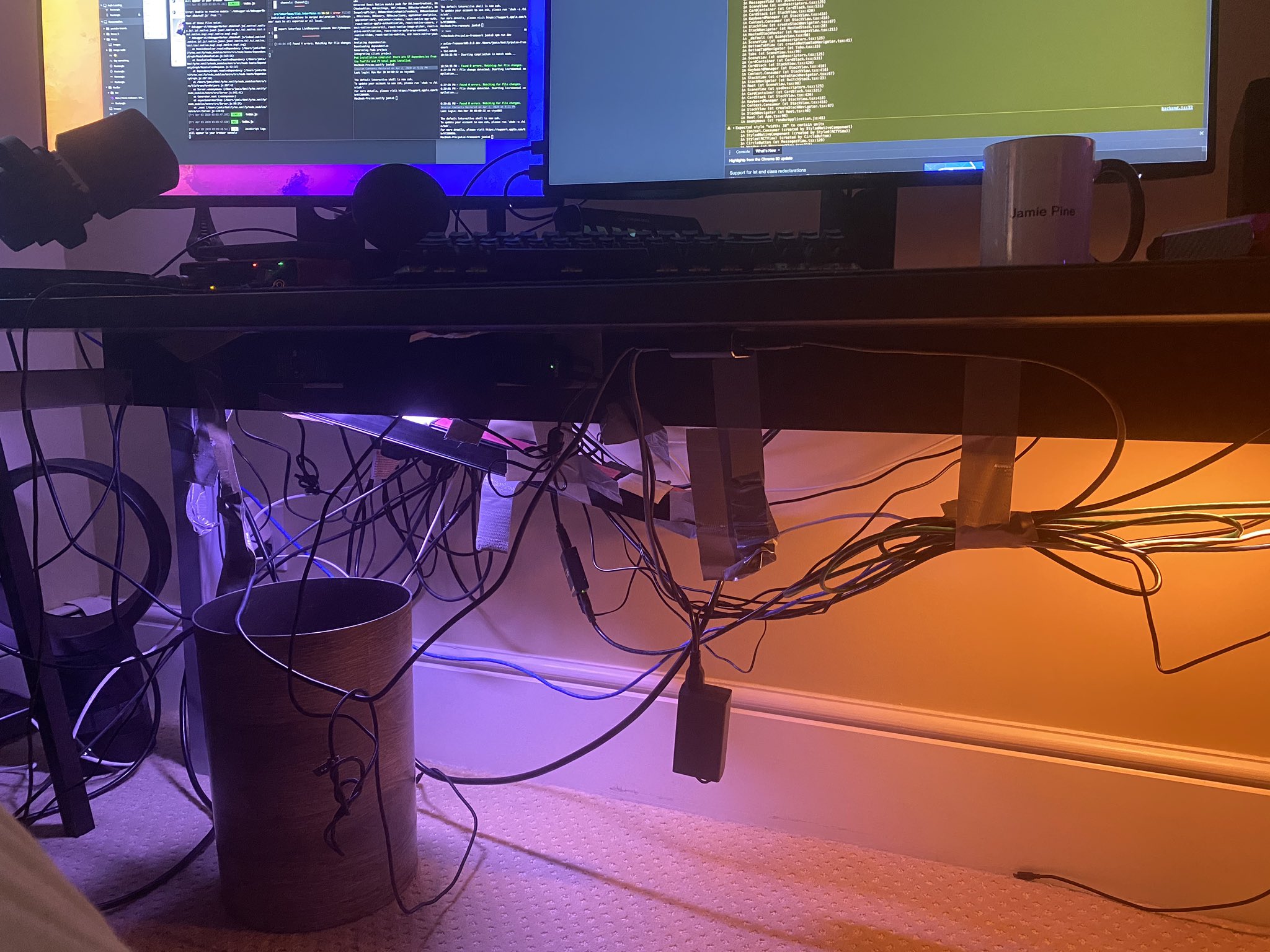
When to use Adapter?
Question: When I need a USB type C Adapter
?
Answer: When you need to connect Printer, Flash or Monitor
to Macbook
, right?
- Having a Macbook, that only have the USB type C port.
- Having incompatible (non-USB type C) devices:
Printer (USB type B)
Flash (USB type A)
Monitor (HDMI)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
| # Input 2: Having incompatible (non-USB type C) devices:
class Printer:
name = "Printer"
def connect_usb_b(self):
return f"{self.name} is connected: Printing..."
# Input 2: Having incompatible (non-USB type C) devices:
class Flash:
name = "Flash"
def connect_usb_a(self):
return f"{self.name} is connected: Copying..."
# Input 2: Having incompatible (non-USB type C) devices:
class Monitor:
name = "Monitor"
def connect_hdmi(self):
return f"{self.name} is connected: Displaying..."
|
Expected Output:
- Making that non-USB type C devices have USB type C port
Printer (USB type C)
Flash (USB type C)
Monitor (USB type C)
- MacBook is able to connect to those incompatible devices
1
2
3
| Printer is connected: Printing...
Flash is connected: Copying...
Monitor is connected: Displaying...
|
How to implement Adapter?
Non-Adapter implementation:
1
2
3
4
5
6
7
8
9
10
11
12
| if __name__ == "__main__":
devices = [Printer(), Flash(), Monitor()]
for device in devices:
if isinstance(device, Printer):
# Expected Output 2: MacBook is able to connect to those incompatible devices
print(device.connect_usb_b())
elif isinstance(device, Flash):
# Expected Output 2: MacBook is able to connect to those incompatible devices
print(device.connect_usb_a())
elif isinstance(device, Monitor):
# Expected Output 2: MacBook is able to connect to those incompatible devices
print(device.connect_hdmi())
|
Adapter Implementation:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
| class Adapter:
def __init__(self, obj, **adapted_methods):
self.obj = obj
self.__dict__.update(adapted_methods)
def __getattr__(self, attr):
return getattr(self.obj, attr)
if __name__ == "__main__":
devices = []
printer = Printer()
# Expected Output 1: Making that non-USB type C devices have USB type C port
devices.append(Adapter(printer, connect=printer.connect_usb_b))
flash = Flash()
# Expected Output 1: Making that non-USB type C devices have USB type C port
devices.append(Adapter(flash, connect=flash.connect_usb_a))
monitor = Monitor()
# Expected Output 1: Making that non-USB type C devices have USB type C port
devices.append(Adapter(monitor, connect=monitor.connect_hdmi))
for device in devices:
# Expected Output 2: MacBook is able to connect to those incompatible devices
print(device.connect())
|
Source Code